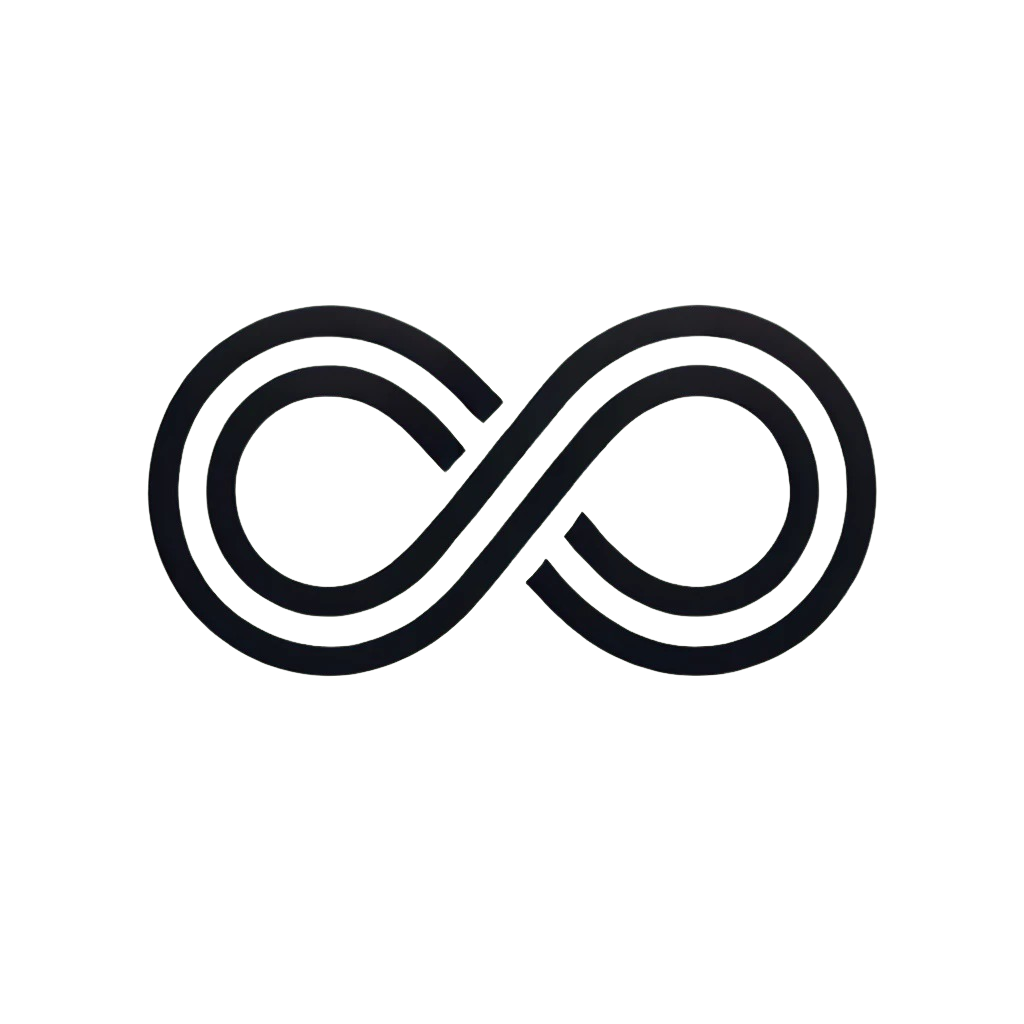
INFINIT ENGINE
A downloadable Engine
Buy Now$60.00 USD or more
Infinit Engine Documentation
Infinit Engine is a lightweight, Python-based engine IDE built on top of Pygame and Tkinter. It enables developers to create games and applications with features like code execution, asset importing, background customization, and compiling Python scripts into standalone executables.
Code Editor
The engine includes an integrated Tkinter-based code editor.
- Features:
- Write, edit, and execute Python scripts.
- Save scripts to files.
- Import image assets for use in games.
- Change background color using a color picker.
- Compile scripts into standalone executables
Functionality
1. Code Execution
Execute Python code directly from the editor.
2. Saving Code
Save editor content to a .py
file.
3. Importing Assets
Import image files and load them into the engine.
Compile scripts into standalone .exe
files using PyInstaller.
python Copy code def compile_to_exe(): compile_command = f"pyinstaller --onefile ..."
Editor Buttons
- Copy & Paste: Edit text in the editor.
- Run Code: Execute Python scripts.
- Save Code: Save scripts to
.py
files. - Import Asset: Add images to the project.
- Settings: Change the background color.
- Compile to EXE: Compile Python scripts to executables.
Usage Instructions
- Run the Engine:
- Write Code:
- Open the editor window.
- Write or paste your Python code.
- Save and Compile:
- Save the script.
- Compile to an executable if needed.
- Compile to other Platforms with other tools. (noy my code, i havent seen the code)
- Pynes
- https://www.google.com/url?sa=t&rct=j&q=&esrc=s&source=web&cd=&cad=rja&uact=8&ve...
Dependencies
- Python 3.x
- Pygame (
pip install pygame
) - Tkinter (bundled with Python)
- PyInstaller (
pip install pyinstaller
) - Optional: Moderngl, Pyopengl, opengl (3D) any python library.
Updated | 19 days ago |
Status | Released |
Category | Tool |
Author | TatorTillInfinity |
Genre | Adventure, Platformer |
Tags | 2D, 3D, 3D Platformer, First-Person, Game engine, Pixel Art, Retro, Singleplayer |
Purchase
Buy Now$60.00 USD or more
In order to download this Engine you must purchase it at or above the minimum price of $60 USD. You will get access to the following files:
infinitengine.exe 29 MB
Development log
- Game StructureJan 26, 2025
- Infinit GraphicsJan 20, 2025
- Theme EditorJan 01, 2025
- Name ChangeDec 23, 2024
- Infinit Engine UpdatesDec 17, 2024
- IE CodeDec 10, 2024